Short introduction to Spring Security Architecture
Short introduction to Spring Security Architecture
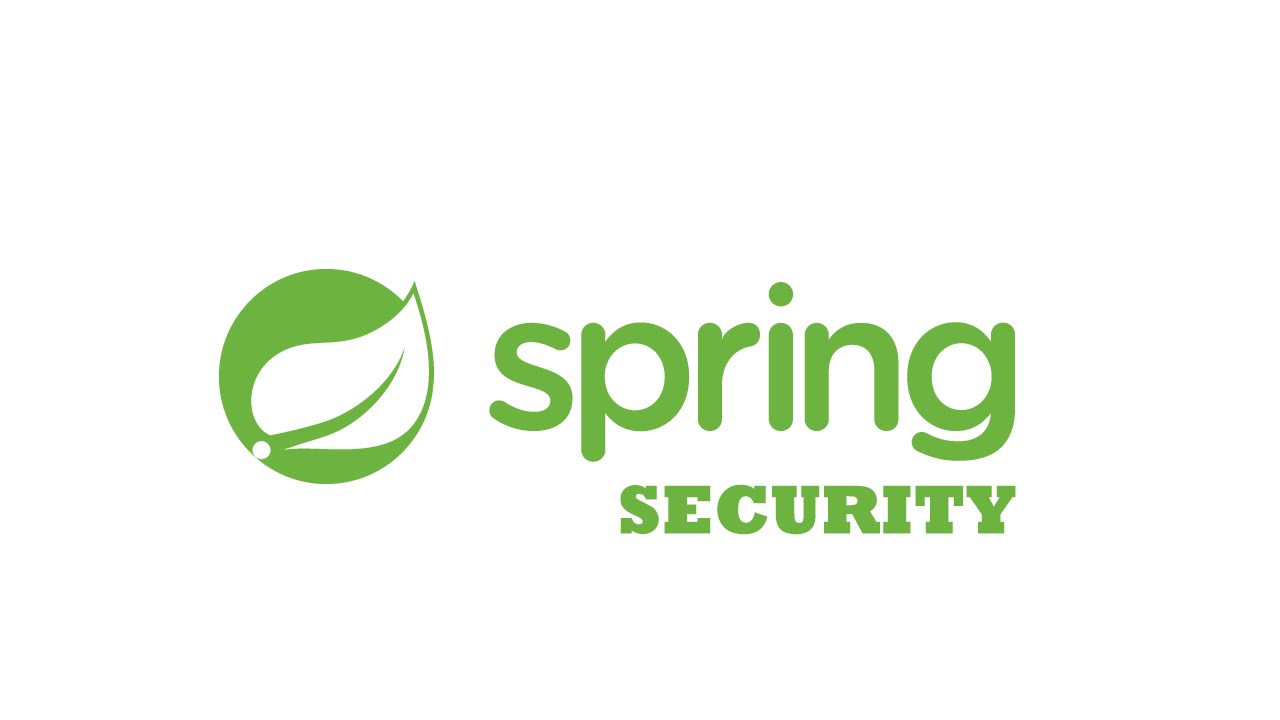
Short introduction, how spring works step by step
1. Authentication Filter
- Http Servlet Request
- Authentication Filter (Extract Username and Password from Header)
- Create Username and Password
"Authentication Token"
"UsernamePasswordAuthenticationToken"
- Pass
"Authentication Token"
to"Authentication Manager"
2. Authentication
After the system is successfully Authenticated the identity, it will return new
"Authenticated Token"
with:
- Principal: UserDetails
- Credentials: (we no longer need to keep the password in our memory because it is not safe)
- Authorities: ROLE_USER
- Authenticated: true
Example: AbstractUserDetailsAuthenticationProvider
protected Authentication createSuccessAuthentication(Object principal,
Authentication authentication, UserDetails user) {
UsernamePasswordAuthenticationToken result = new UsernamePasswordAuthenticationToken(
principal, authentication.getCredentials(),
authoritiesMapper.mapAuthorities(user.getAuthorities()));
result.setDetails(authentication.getDetails());
return result;
}
UsernamePasswordAuthenticationToken
public UsernamePasswordAuthenticationToken(Object principal, Object credentials,
Collection<? extends GrantedAuthority> authorities) {
super(authorities);
this.principal = principal;
this.credentials = credentials;
super.setAuthenticated(true);
}
3. AuthenticationProvider and UserDetails/Service
"Authentication Manager"
delegate "Authenticated Token"
to "Authentication Provider"
More detailed:
ProviderManager is default implementation of interface Authentication Manager, when we delegate providers to ProviderManager, it will check all providers, are they are supported or not, it will only use those providers that are supported.
P.S: "Authentication Manager"
is interface and "ProviderManager"
is default implementation of this interface.
4. Security Context - it is holder for context information, related to security.
To access protected endpoints, you need to store "Authentication Token"
that will be easily accessible,
that’s where the "ThreadLocal"
comes in, but we don’t use it directly,
we use a higher level wrapper, "Security Context"
.
To save "Authentication Token"
some where, we use "Security Context"
which allow us to stores a list of rules "SecurityContext"
use ThreadLocal<SecurityContext>
inside implementation.
"ThreadLocal"
- allows us to store data that will be accessible only by a specific thread,
each thread holds it’s own copy of data, as long as thread is alive.
Authentication Recap
- “Authentication Filter” - creates and “Authentication Token” and passes it to the “Authentication Manager”.
- “Authentication Manager” - delegates to the “Authentication Provider”.
- “Authentication Provider” - uses a “UserDetailsService” to load the “UserDetails” and returns an “Authenticated Principal”, back to the “Authentication Manager”.
- “Authentication Manager” - pass back to “Authentication Filter”.
- “Authentication Filter” - sets the “Authentication Token” to the “SecurityContext”.
5. Filter Security Interceptor - last filter in security filter chain, that protect access to protected resource
At the last stage, the authorization is based on the url of the request. FilterSecurityInterceptor is inherited from AbstractSecurityInterceptor and decides, does current user has access to the current url.
Authorization Recap
- FilterSecurityInterceptor obtains the “Security Metadata” by matching on the current request
- FilterSecurityInterceptor gets the current “Authentication”
- The “Authentication”, “Security Metadata” and Request is passed to the “AccessDecisionManager”
- The “AccessDecisionManager” delegates to it’s “AccessDecisionVoter(s)” for decision.
6. Access Decision
7. Access Denied
Exception Handling Recap
When “Access Denied” for current Authentication, the ExceptionTranslationFilter delegates to the AccessDeniedHandler, which by default, returns a 403 Status.
When current Authentication is “Anonymous”, the ExceptionTranslationFilter delegates to the AuthenticationEntryPoint to start the Authentication process.
Books:
More detailed articles:
- https://habr.com/ru/post/346628/
- https://spring.io/guides/topicals/spring-security-architecture/
- https://www.baeldung.com/java-threadlocal
- https://docs.oracle.com/javase/7/docs/api/java/lang/ThreadLocal.html
- https://drive.google.com/file/d/1uiOKOxSI33AxW4EXpzXwbj0z77zJk0Ev/view
- https://docs.spring.io/spring-security/site/docs/5.2.x/reference/htmlsingle/#filter-ordering
- https://gist.github.com/zmts/802dc9c3510d79fd40f9dc38a12bccfc
Other articles:
- http://it-uroki.ru/uroki/bezopasnost/identifikaciya-autentifikaciya-avtorizaciya.html
- https://auth0.com/blog/implementing-jwt-authentication-on-spring-boot/
Share this post